Learning C++ in University is a lot more in-depth than learning snippets of code online. The first week of school involved almost no coding at all, but instead focused on the various things you need to know in order to begin programming. Today I am here to share a bit about what those things are AND help you write your first program. The best part, is that you'll also know what everything does!
Memory Usage
One thing that may look difficult when you begin, is attempting to decipher all of the Syntax (language rules) for the type of programming you want to code. The best way to think of it is like grammar. There are different ways to put together a sentence, but only some of them will make sense. You can know what each part is, but without the correct order—your computer can't read it.
The next hurdle is understanding that each datatype stores a set amount of information. This way the computer running the application knows exactly how much space to store for each variable and object in the computer's RAM (random access memory). The reason why this is important is so that the system knows where each thing is and can find them with memory addresses when your program needs them. You can think of memory addresses as a physical address where each variable lives!
On top of being able to create custom datatypes in C++, here are some of the basic ones to help you visualize how much space each one takes up. This way, you can write applications that are efficient and run quickly.
one byte = 8 bits of information.
- char (one character or symbol 'A', 'a', '$') all take up one byte
- bool (true or false/ 1 or 0) takes up one byte
- int (integer/ whole number) Depending on how large it is, can take up to 64 bytes
Then we have things like long (long integers, double (with decimal points) and float numbers) which take up significantly more space. Knowing which one to use where, allows your program to use only the resources it needs.
The Headers
In each application, you must include the libraries that your program needs for the types of data it will be using. The header always precedes main. Some of these are:
#include<iostream>
(which includes I/O to the console screen. Like receiving data from a user, and typing it to the screen. You will obviously need this one!)
#include<string>
(to read and write string functions and sentences)
#include
tells the compiler to include the library that follows it.
After the header, you must include:
using namespace std;
(This is using the standard namespace)
The Main Function
In every C++ application, you must include a main function in order for it to work. I just call it "main". You can think of main, as the main part of the program—where it begins. There are 3 parts for every function. The datatype of what it's supposed to return (int for integer value), the name ("main") and the parentheses, to put external variables in that the function uses. To write a basic main function looks like this:
int main()
{
return 0;
}
In between the curly brackets, you put your statements (individual instructions like sentences) for your function/module to carry out. You need to use them or the body of your function won't run. You will soon learn that for every open bracket, you'll need to close them too! When starting programming, we leave return as 0 (which is an integer), to say that the program finished without problems. It returns the value zero.
Words That You Will See in Code
cout
(console out) writing out to screen. <<
always goes before AND after a cout statement.
cin
(console in) receive user input. >>
always follows the word cin.
endl
end the line. I call it "end el"!
- also, any sentence/string that goes in or out "must be inside quotes!"
I literally call these "cout" and "sin", to remember them easily. As long as you know what it does, what you call them doesn't matter as much. At the end of a statement, you always put a semicolon ;
to execute the statement. What I found entertaining was that char
can be called "char", "care", or "car", and everyone will know what you're talking about.
Declaring A Variable
Declaring a variable is 3 parts (variables are what the statements manipulate)
- The datatype the variable is
- it's name (first word lowercase, second and on start at upper-case. Not mandatory, but conventional)
- And if it starts with a value, (initializing a variable with a value) you state what it is (example: x = 0)
- semicolon (because announcing a variable IS a statement!)
A Very Simple Program
This program will ask for a users name, then greet them. No "Hello world" for us! You will need a compiler to run it, but if you don't want to download something, you can try it on cpp.sh which is an online compiler. Cpp meaning "c++" and sh, meaning "shell". When you use a compiler program your file will save as whateverYouNameIt.cpp.
#include<iostream>
#include<string>
using namespace std;
int main()
{
string yourName;
cout << "What is your name?" << endl;
cin >> yourName;
cout << "Hello " << yourName << endl;
return 0;
}
- Include the I/O stream
- Include string libraries
- use standard namespace
- Main function header
- Opening bracket
- Announce a string variable called "yourName"
- Print to screen "What is your name?"
- Receive user input for yourName
- Print out greeting with yourName that the user entered
- Return 0
- Closing bracket
Learn C++ With Shello! Series:
If you missed something prior to this tutorial, make sure to check out the other post! I will be adding more tutorials coming up!
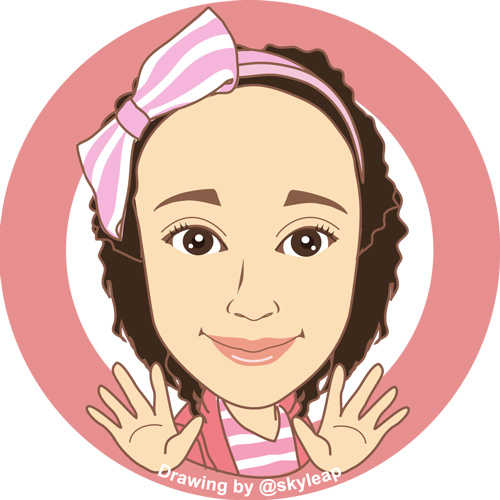
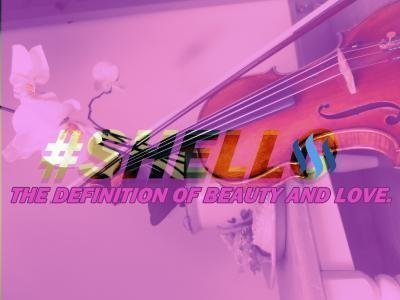
Thank you @bollutech for this art!

I'm designing a text-based game from scratch!
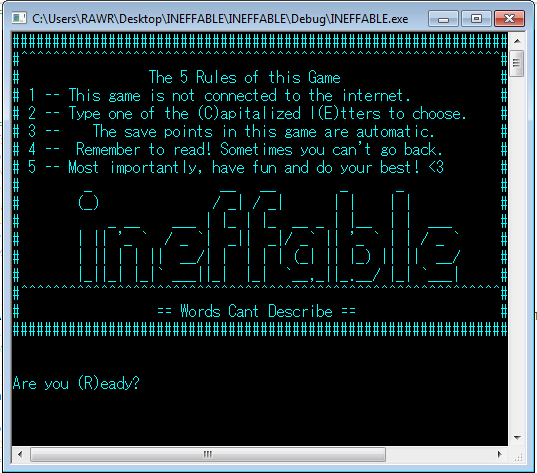
Some of the things I will be covering coming up
will include video game mechanics!~
Stay tuned! 💞
Thanks for this @shello, I recently delved into the world of coding but my major challenge has been staying consistent. There is too much distraction around
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Yeah, consistency is key. I'm lucky that I'm doing this outside of my free time. Keep it up, and I know you'll get it. Thanks for stopping by @tojukaka!~ :D
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
nice! I passed this on to some friends
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thankuu <3 <3 <3
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I don't know why, but I just love, love, LOVE the way you explain things! It's simple, concise but descriptive too.
I have been attempting to teach myself C++ and it has been a nightmare (which is a crying shame because me dad was a computer programmer for over 30 years and taught me the basics). I am horrible at writing out proper syntax (ToT)-- I frequently forget commands.
It also doesn't help that my compiler is a piece of s*** that seems to hate my increasingly antiquated Dell. Oi vey!
At any rate keep those tutorials coming, I hope to learn more of what I missed during my lessons. Stay cool Shello!
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you @puddinpaws, the love is much appreciated! 💝
My compiler isn't the best either (I should really update). It requires additional libraries that aren't required in newer versions. Wow, you already have strong roots in programming—hats off to you!~
I also tried to learn C++ before I had school for it, and tbh, reading through forums is only useful now that I can grasp and understand the fundamentals. My goal is always try to explain in the simplest way possible, it makes learning so much more fun!
I shall keep the tutorials incoming (between my other "normal" posts of course), I genuinely want to see more Steemians pick up coding. I feel it would help the future of blockchain :D
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit