One of the most frequently in Android App Development is a login form and signup. When the user is valid, then the user will be redirect to another page. And there is option when the user want to signup first. Typically, the user will be redirect to a listing page. Today, i will show you how to implement product listing app with signup and login form for view and binding to java code.
I assumed you have read my post before how to install android studio, Java, jdk and other things that need to implement this project.
Get started with the MainActivity and layout
First of all, you need make the layout for the login form. In this case, we make the xml file in the activity_main.xml. Copy this code below. It's up to you if you don't want it is in activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true">
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingTop="56dp"
android:paddingLeft="24dp"
android:paddingRight="24dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="24dp"
android:textSize="24sp"
android:text="PRODUCT LISTING"
android:layout_gravity="center_horizontal" />
(html comment removed: Email Label )
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp">
<EditText android:id="@+id/input_email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:hint="Email" />
</android.support.design.widget.TextInputLayout>
(html comment removed: Password Label )
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp">
<EditText android:id="@+id/input_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:hint="Password"/>
</android.support.design.widget.TextInputLayout>
<android.support.v7.widget.AppCompatButton
android:id="@+id/btn_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:textColor="@color/colorWhite"
android:layout_marginBottom="24dp"
android:padding="12dp"
android:text="Login"/>
<TextView android:id="@+id/signup_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="24dp"
android:text="Don't have an account? Sign Up"
android:gravity="center"
android:textSize="16dip"/>
</LinearLayout>
</ScrollView>
The above xml describe that you have 2 Label, 2 TextInputLayout and 1 Login Button. When the user clicks the Login Button, it will redirect to the product listing page. It hasn’t validate if the user is valid or not. You can validate from your own needs.
And in the login there is signup option via text. So, we need to make our layout and its java class though. This is the signup layout xml file. I named it activity_signup.xml
<?xml version="1.0" encoding="utf-8"?>
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true">
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingTop="56dp"
android:paddingLeft="24dp"
android:paddingRight="24dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="24sp"
android:text="@string/product_listing"
android:layout_marginBottom="24dp"
android:layout_gravity="center_horizontal" />
(html comment removed: Name Label )
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp">
<EditText android:id="@+id/input_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textCapWords"
android:hint="@string/name" />
</android.support.design.widget.TextInputLayout>
(html comment removed: Email Label )
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp">
<EditText android:id="@+id/input_email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:hint="@string/email" />
</android.support.design.widget.TextInputLayout>
(html comment removed: Password Label )
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp">
<EditText android:id="@+id/input_password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:hint="@string/password"/>
</android.support.design.widget.TextInputLayout>
(html comment removed: Signup Button )
<android.support.v7.widget.AppCompatButton
android:id="@+id/btn_signup"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="24dp"
android:layout_marginBottom="24dp"
android:background="@color/colorPrimary"
android:textColor="@color/colorWhite"
android:padding="12dp"
android:text="@string/create_account"/>
<TextView android:id="@+id/login_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="24dp"
android:text="@string/account_desc"
android:gravity="center"
android:textSize="16sp"/>
</LinearLayout>
</ScrollView>
This is the result for building login and signup page
Login Page
SignUp Page
Binding to Java Class
You can use designer from android studio to drag and drop to make the same layout. But, i made this via xml manually. Then, you have to make the Java code to interact with the user and binding from layout xml to the code. You have to add the listener to the button to redirect to the new page. And if you want to validate, you must bind the username input and password input to your java code and manipulate with them. In this login page, there is also option to go to signup page if you want to. But, this tutorial purpose is to learning about view and binding it to java code. I am using Butterknife to bind and set onclicklistener to the views.
package upwork.andri.productlisting;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import butterknife.ButterKnife;
import butterknife.OnClick;
public class MainActivity extends AppCompatActivity {
@OnClick(R.id.btn_login) void login(){
Intent intent = new Intent(this, ProductListingActivity.class);
startActivity(intent);
}
@OnClick(R.id.signup_text) void goToSignUp(){
Intent intent = new Intent(this, SignUpActivity.class);
startActivity(intent);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
}
}
And as i said before we need the SignupActivity.java to bind from xml too. This is it.
package upwork.andri.productlisting;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.widget.Toast;
import butterknife.ButterKnife;
import butterknife.OnClick;
import butterknife.Unbinder;
public class SignUpActivity extends AppCompatActivity {
private Unbinder unbinder;
@OnClick(R.id.btn_signup) void SignUp(){
Toast.makeText(this, "Sign up clicked", Toast.LENGTH_SHORT).show();
}
@OnClick(R.id.login_text) void goToLogin(){
Intent intent = new Intent(this, MainActivity.class);
startActivity(intent);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_signup);
unbinder = ButterKnife.bind(this);
}
@Override
protected void onDestroy() {
super.onDestroy();
unbinder.unbind();
}
}
Connect to Product Listing Activity
The Product Listing App use the Activity to contain the view inside it. You can made it via fragment. It is using RecyclerView. You can copy below the layout. Very simple.
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.RecyclerView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/rv_item_product"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layoutManager="android.support.v7.widget.LinearLayoutManager">
</android.support.v7.widget.RecyclerView>
And this is where we connect to the ProductListingActivity.java
package upwork.andri.productlisting;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.RecyclerView;
import butterknife.BindView;
import butterknife.ButterKnife;
import butterknife.Unbinder;
import upwork.andri.productlisting.model.Product;
public class ProductListingActivity extends AppCompatActivity {
private Unbinder unbinder;
@BindView(R.id.rv_item_product)
protected RecyclerView mItemProductRV;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_product_listing);
unbinder = ButterKnife.bind(this);
ProductAdapter itemOrderAdapter = new ProductAdapter(this, Product.createProductItems());
mItemProductRV.setAdapter(itemOrderAdapter);
}
@Override
protected void onDestroy() {
super.onDestroy();
unbinder.unbind();
}
}
And, this is the Product Listing Result :)
Proof of work
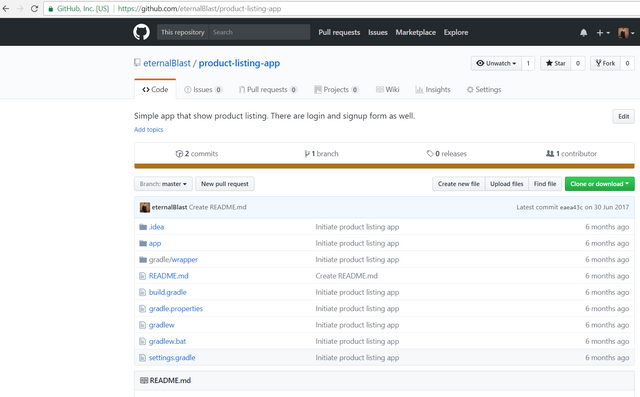
You can play around with the code and open up pull request to add feature or validation if you would want. You can view it on this link.
https://github.com/eternalBlast/product-listing-app
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Smoga approve :)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Makasih bang :)
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @andrixyz I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit