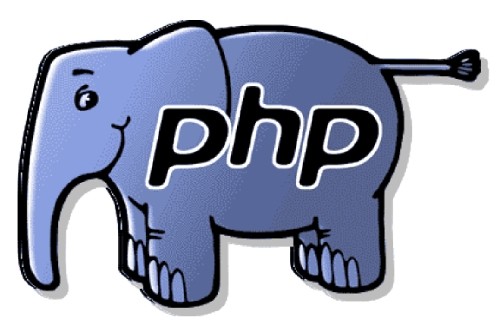
What Will I Learn?
This tutorial is all about how to use null coalescing operator in PHP7.
- What is null coalescing operator
- How to use null coalescing operator
- What is the difference between null coalescing and ternary operator
Requirements
- PHP 7 Server
- Basic knowledge about PHP
Difficulty
- Basic
Tutorial Contents
What is Null Coalescing operator?
The null coalescing operator (??) will check if the first operand is set or not NULL. If it is not NULL, then it will return the value of the first operand. Otherwise, it will return the value of the second operand.
How to use null coalescing operator?
This is the syntax for null coalescing operator:
$c = $a ?? $b;
Example:
<?php
$a = 'String A';
$b = 'String B';
$c = $a ?? $b;
echo $c;
?>
This example will output 'String A' because variable $a
has a value.
Another Examples:
<?php
$a = NULL;
$b = 'String B';
$c = $a ?? $b;
echo $c; // This will output 'String B';
?>
<?php
$b = 'String B';
$c = $a ?? $b;
echo $c; // This will output 'String B';
?>
But if variable $a
is equal to NULL or UNDEFINED, then it will output 'String B'.
<?php
$a = '';
$b = 'String B';
$c = $a ?? $b;
echo $c; // This will output an empty string;
?>
But if you set variable $a
into an empty string, then it will return variable $a
(empty string).
<?php
$d = 'String D';
$e = $a ?? $b ?? $c ?? $d;
?>
You can also have multiple null coalescing operator in one statement. So in this example, it will output 'String D' because all the previous variables are UNDEFINED.
What is the difference between null coalescing and ternary operator?
The difference between this two operators is that the null coalescing operator evaluates the first operand if it is null. It's like using the isset()
function. While the ternary operator evaluates the first operand as a boolean expression.
Examples:
<?php
$a = '';
$b = 'String B';
echo $a ?? $b; // null coalescing operator
echo $a ?: $b; // ternarty operator
?>
For an empty string, the null coalescing operator will output an empty string because variable $a
is not null. While the ternary operator will output 'String B' because empty string is false.
<?php
$b = 'String B';
echo $a ?? $b; // null coalescing operator
echo $a ?: $b; // ternarty operator
?>
For UNDEFINED variable, the null coalescing operator will output 'String B'. While the ternary operator will throw an undefined variable error.
<?php
$b = false; // same with 0
echo $a ?? $b; // null coalescing operator
echo $a ?: $b; // ternarty operator
?>
For false/0 value, the null coalescing operator will return the exact value (false/0). While the ternary operator will output 'String B'.
So, the difference between the two is that the null coalescing operator will handle the NULL and UNDEFINED variables. While the ternary operator is th shorthand for if-else. Therefore, Null Coalescing operator is not meant to replace the ternary operator.
Curriculum
Posted on Utopian.io - Rewarding Open Source Contributors
@gentlemanoi, No matter approved or not, I upvote and support you.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Thank you for the contribution. It has been approved.
isset()
equivalent of the feature. like:You can contact us on Discord.
[utopian-moderator]
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
I'll do it next time. Thank you so much.
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Hey @gentlemanoi I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit
Nice article :)
https://steemit.com/coding/@gaottantacinque/rasmus-lerdorf-php-inventor-in-milan
Downvoting a post can decrease pending rewards and make it less visible. Common reasons:
Submit